To delete records in the database, following these steps. We have named our Yii2 folder as delete.
Step 1 Create Model file
Create a model file child.php in frontend/models folder.
- <?php
- namespace app\models;
-
- use Yii;
-
- class Child extends \yii\db\ActiveRecord
- {
-
-
-
- public static function tableName()
- {
- return 'child';
- }
-
-
-
-
- public function rules()
- {
- return [
- [['name', 'meaning', 'gender'], 'required'],
- [['name', 'meaning'], 'string', 'max' => 100],
- [['gender'], 'string', 'max' => 15]
- ];
- }
- }
Step 2 Create action in controllers
Create an action actionDelete in ChildController.php file.
- <?php
- namespace frontend\controllers;
-
- use Yii;
- use app\models\Child;
- use yii\web\Controller;
-
-
-
-
-
- class ChildController extends Controller
- {
-
-
-
- public function actionCreate()
- {
-
- $model = new Child();
-
-
- if($model->load(Yii::$app->request->post()) && $model->save()){
- return $this->redirect(['index']);
- }
-
- return $this->render('create', ['model' => $model]);
- }
-
-
-
-
- public function actionIndex()
- {
- $child = Child::find()->all();
-
- return $this->render('index', ['model' => $child]);
- }
-
-
-
-
-
- public function actionEdit($id)
- {
- $model = Child::find()->where(['id' => $id])->one();
-
-
- if($model === null)
- throw new NotFoundHttpException('The requested page does not exist.');
-
-
- if($model->load(Yii::$app->request->post()) && $model->save()){
- return $this->redirect(['index']);
- }
-
- return $this->render('edit', ['model' => $model]);
- }
-
-
-
-
-
- public function actionDelete($id)
- {
- $model = Child::findOne($id);
-
-
- if($model === null)
- throw new NotFoundHttpException('The requested page does not exist.');
-
-
- $model->delete();
-
- return $this->redirect(['index']);
- }
- }
Step 3 Run it
Run it on the browser.
http://localhost/delete/frontend/web/index.php?r=child%2Findex
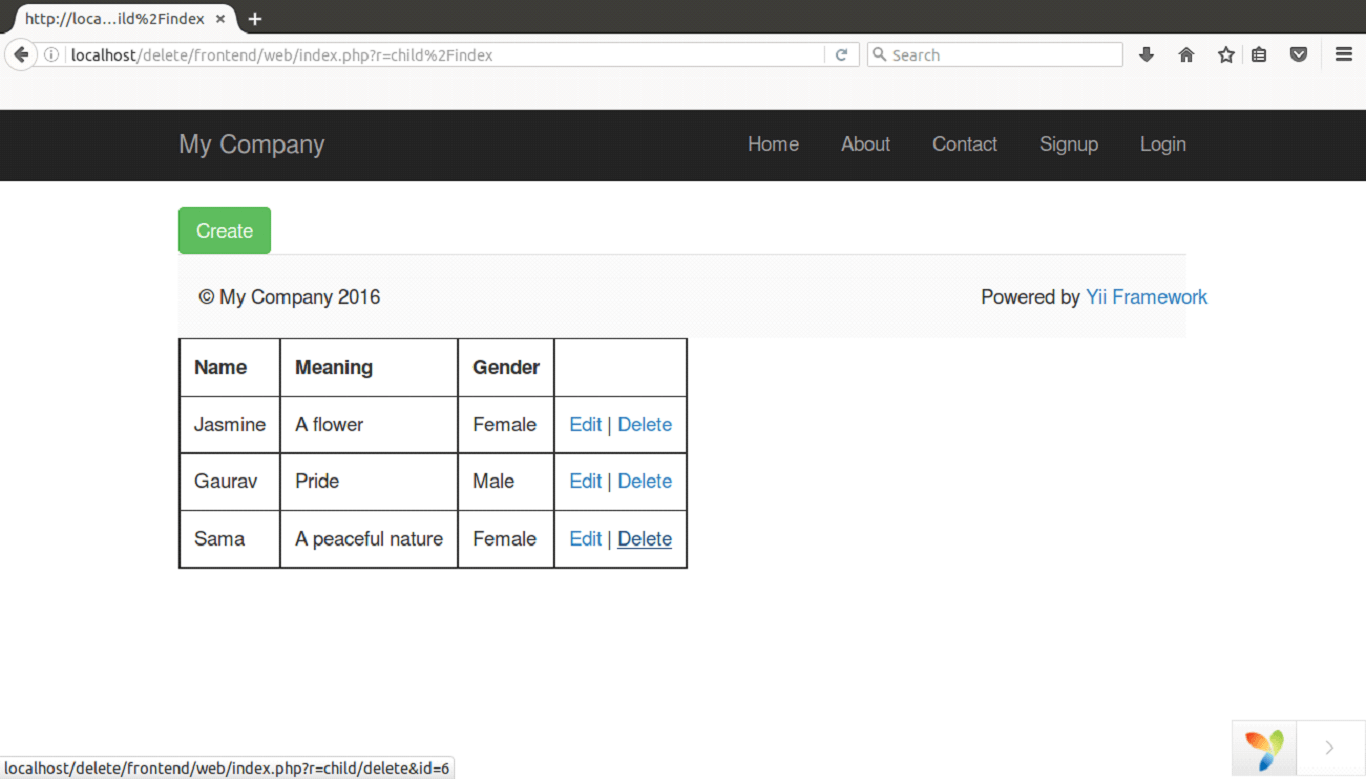
Look at the above snapshot, click the Delete option in the last row of the table.
Look at the above snapshot, respective row is deleted from the table.
0 Comments