To update the records in the database, following these steps. We have named our Yii2 folder as update.
Step 1 Create Model file
Create a model file child.php in frontend/models folder.
- <?php
- namespace app\models;
-
- use Yii;
-
- class Child extends \yii\db\ActiveRecord
- {
-
-
-
- public static function tableName()
- {
- return 'child';
- }
-
-
-
-
- public function rules()
- {
- return [
- [['name', 'meaning', 'gender'], 'required'],
- [['name', 'meaning'], 'string', 'max' => 100],
- [['gender'], 'string', 'max' => 15]
- ];
- }
- }
Step 2 Add action in controllers
Add the update action actionEdit in controllers file ChildController.php.
- <?php
- namespace frontend\controllers;
-
- use Yii;
- use app\models\Child;
- use yii\web\Controller;
-
-
-
-
-
- class ChildController extends Controller
- {
-
-
-
- public function actionCreate()
- {
-
- $model = new Child();
-
-
- if($model->load(Yii::$app->request->post()) && $model->save()){
- return $this->redirect(['index']);
- }
-
- return $this->render('create', ['model' => $model]);
- }
-
-
-
-
- public function actionIndex()
- {
- $child = Child::find()->all();
-
- return $this->render('index', ['model' => $child]);
- }
-
-
-
-
-
- public function actionEdit($id)
- {
- $model = Child::find()->where(['id' => $id])->one();
-
-
- if($model === null)
- throw new NotFoundHttpException('The requested page does not exist.');
-
-
- if($model->load(Yii::$app->request->post()) && $model->save()){
- return $this->redirect(['index']);
- }
-
- return $this->render('edit', ['model' => $model]);
- }
- }
Step 3 Create View file
Create a file edit.php in frontend/view/child folder.
- <?= $this->render('child_view', [
- 'model' => $model,
- ]) ?>
Step 4 Run it
Run it on the browser.
http://localhost/dbb/frontend/web/index.php?r=child%2Fedit&id=6
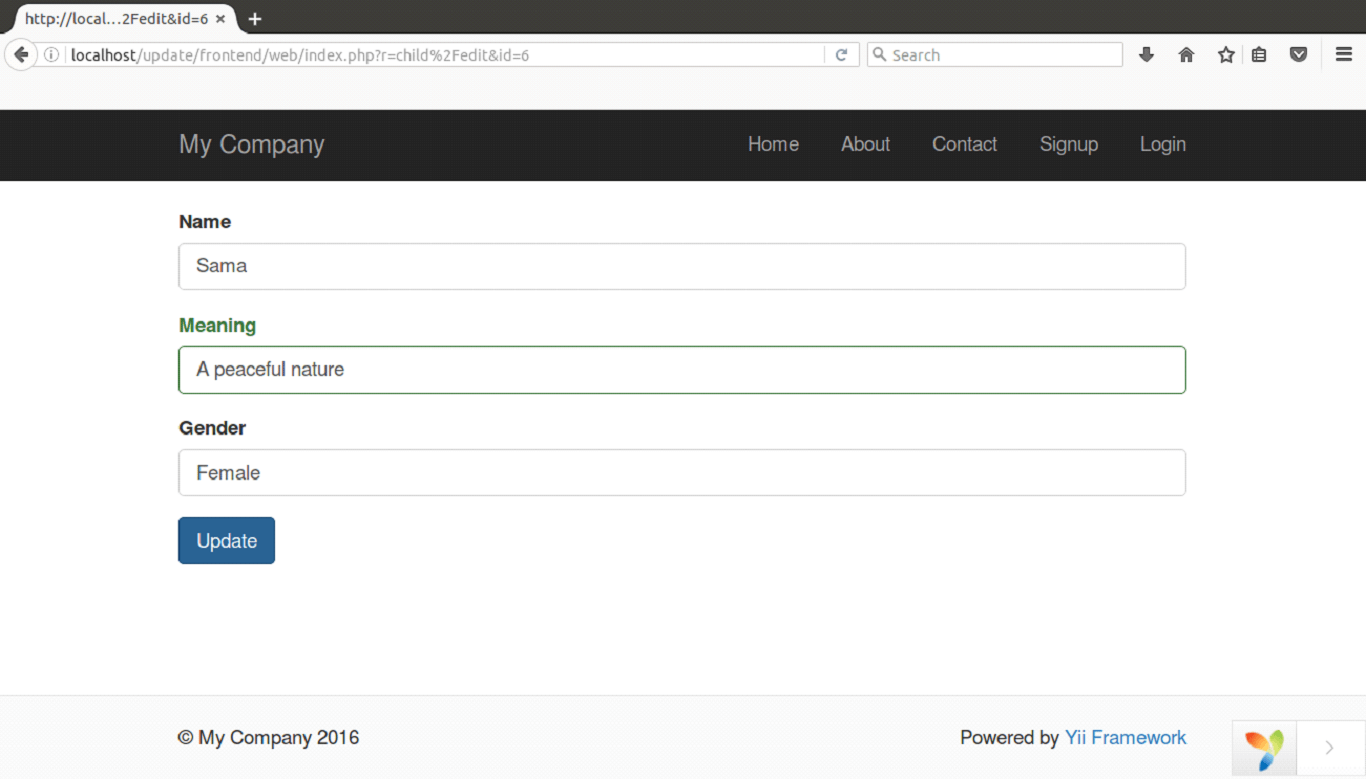
Click on Update, information will be updated.
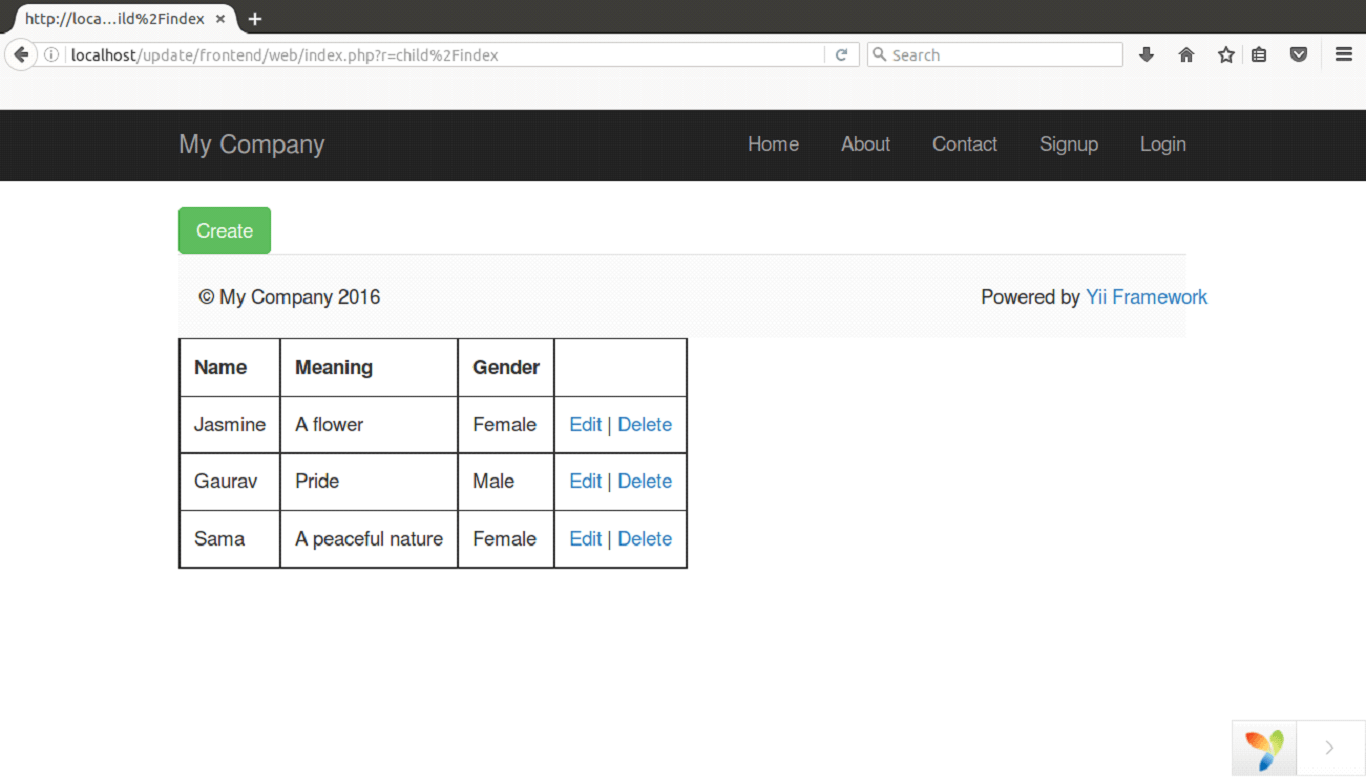
Look at the last row in above snapshot, data is updated.
0 Comments